Prompt Is Not Defined Error in JavaScript
When working with JavaScript, it is common to encounter errors that can be challenging to debug. One such error is the “Prompt Is Not Defined” error. This error occurs when using the prompt()
function, which is a built-in method in JavaScript for requesting user input. This article will explore the causes and solutions for this error, helping you overcome it and improve your JavaScript coding skills.
Key Takeaways
- The “Prompt Is Not Defined” error occurs when using the
prompt()
function in JavaScript. - This error often arises due to the incorrect use of the
prompt()
function or missing browser compatibility. - To resolve this error, ensure that the
prompt()
function is correctly implemented and consider using alternative methods for user input.
Understanding the “Prompt Is Not Defined” Error
When you encounter the “Prompt Is Not Defined” error in your JavaScript code, it means that the prompt()
function is not recognized by the JavaScript interpreter. This error typically occurs when:
- You misspell
prompt
aspromptt
or some other variation. - You forget to include parentheses after
prompt
(e.g.,prompt()
). - Your code is running in an environment where the
prompt()
function is not supported, such as in a server-side JavaScript environment like Node.js.
It is important to double-check your code for any spelling mistakes or missed parentheses when using the prompt()
function. Additionally, be aware of the particular environment you are working in and whether it supports the prompt()
function.
Common Causes and Solutions
1. Misspelled or Incorrect Usage of prompt()
One of the common causes of the “Prompt Is Not Defined” error is misspelling or incorrect usage of the prompt()
function. Make sure you have spelled prompt
correctly, and remember to include the parentheses after it. Correct usage: prompt()
.
Remember: Even a small typo in the function name can result in the error, so pay close attention to the syntax.
2. Browser Compatibility
Another common cause of this error is incompatible browser support. The prompt
function is primarily supported by web browsers, so if you are using JavaScript in a server-side environment like Node.js, the function might not be available.
To resolve this, you can consider using alternative methods for user input, such as HTML input fields, form submission, or libraries that provide custom modal windows.
Note: Always check the browser compatibility of any JavaScript function you intend to use to ensure it is supported.
3. External JavaScript Files
If you are using an external JavaScript file in your HTML code, make sure to include the script tag correctly and ensure the file is located in the correct directory. Failure to load the external file can lead to the “Prompt Is Not Defined” error.
Interesting fact: Use the Developer Tools in modern browsers to check if the external JS file is being loaded without any errors.
Examples and Solutions
Here are a few examples that illustrate how to implement the prompt()
function correctly:
Example | Correct Usage |
---|---|
Example 1 | var name = prompt('Please enter your name:'); |
Example 2 | var age = Number(prompt('Please enter your age:')); |
Interesting fact: It is possible to convert the returned string from prompt()
to a specific data type or perform additional operations on it.
If you are still encountering the “Prompt Is Not Defined” error, consider the following solutions:
- Check for any misspellings or incorrect usage of the
prompt()
function in your code. - Ensure that the browser you are using supports the
prompt()
function. - If using an external JavaScript file, double-check that the file is properly included in your HTML and located in the correct directory.
- Explore alternative methods for user input if the
prompt()
function is not available in your runtime environment.
Conclusion
The “Prompt Is Not Defined” error can be easily resolved by checking for misspellings, ensuring correct usage of the prompt()
function, and considering browser compatibility. Remember to double-check your code and explore alternative methods if necessary to avoid this error.
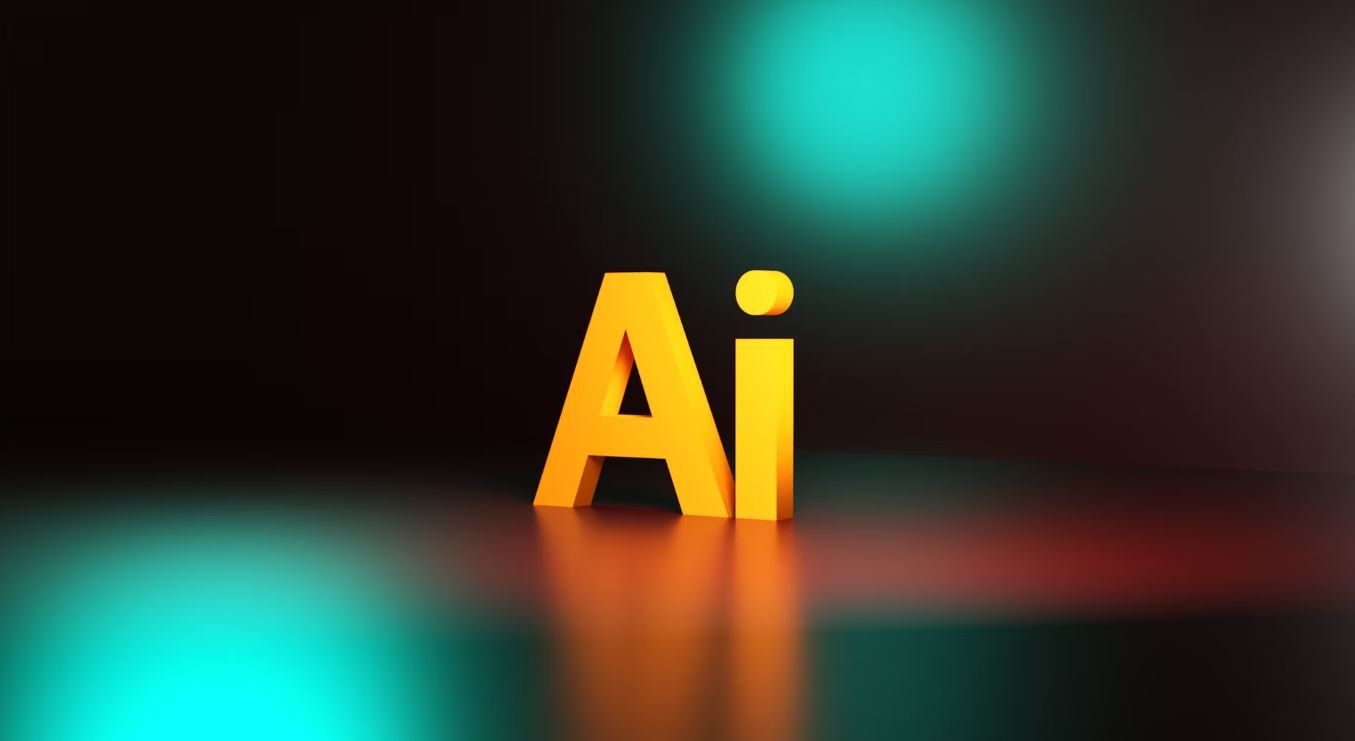
Common Misconceptions
Misconception 1: prompt is not defined error is related to syntax errors in JavaScript
One common misconception is that the “prompt is not defined” error in JavaScript is caused by syntax errors in the code. While syntax errors can lead to exceptions and errors in JavaScript, this error specifically occurs when the prompt
function is used in a non-supported environment.
- The “prompt is not defined” error typically occurs in non-browser environments.
- It can also occur when working with JavaScript tools or frameworks that do not provide access to the
prompt
function. - This error can be resolved by using alternative methods to collect user input in non-supported environments.
Misconception 2: The prompt is not defined error is caused by a missing import or script reference
Another misconception is that the “prompt is not defined” error is the result of a missing import or script reference in the HTML file. While missing or incorrect imports can cause other types of errors, the “prompt is not defined” error specifically occurs when the prompt
function is not available.
- The error can occur if the JavaScript file that uses the
prompt
function is not properly included or referenced in the HTML file. - It is important to ensure that the necessary JavaScript files are correctly referenced in the HTML file to avoid this error.
- However, a missing or incorrect import or script reference alone will not cause the “prompt is not defined” error.
Misconception 3: All modern browsers support the prompt function
Many people assume that all modern browsers support the prompt
function in JavaScript, but this is not entirely accurate. While most popular browsers do support the prompt
function, there are scenarios where it may not be available.
- Some mobile browsers or embedded browsers in certain devices may not support the
prompt
function. - Browser extensions or add-ons can potentially disable or restrict the functionality of the
prompt
function. - It is essential to consider the target environment and browser compatibility when developing JavaScript code that relies on the
prompt
function.
Misconception 4: Disabling JavaScript results in the prompt is not defined error
Another common misconception is that if JavaScript is disabled in the browser settings, the “prompt is not defined” error will occur. However, this error specifically relates to the availability of the prompt
function, regardless of whether or not JavaScript is enabled or disabled.
- Disabling JavaScript will prevent the execution of all JavaScript code, including any code that relies on the
prompt
function. - The “prompt is not defined” error can occur even if JavaScript is enabled if the environment or framework does not provide access to the
prompt
function. - Disabling JavaScript would simply prevent any JavaScript code, including the
prompt
function, from running in the browser.
Misconception 5: The prompt is not defined error is a critical issue that should always be fixed
It is a misconception to believe that the “prompt is not defined” error is always a critical issue that needs to be fixed. While the error can cause unexpected behavior if the prompt
function is essential for the functionality, it does not necessarily indicate a severe problem.
- If the
prompt
function is not critical for the operation of the JavaScript code, the error can be ignored or handled gracefully. - In some cases, it may be appropriate to provide alternative input methods or fallback options to handle the absence of the
prompt
function and prevent the error from interrupting the program flow. - It is important to assess the impact of the error and decide whether fixing the absence of the
prompt
function is necessary or if alternative solutions can be implemented.
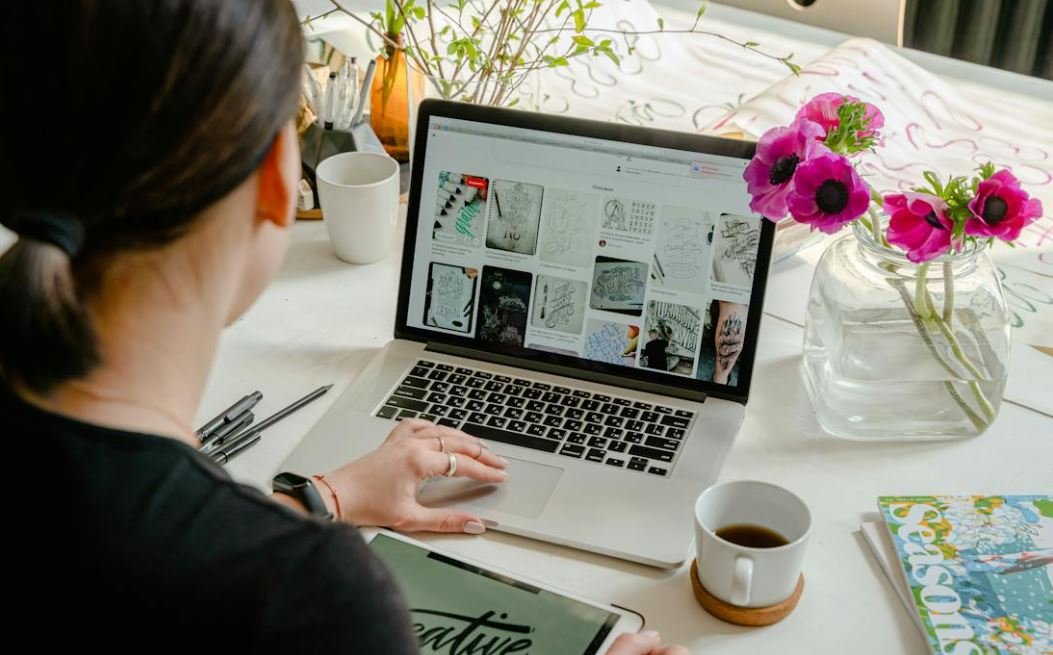
The Concept of Prompts in JavaScript
In JavaScript, prompts are used to interact with users by requesting input. However, sometimes you may encounter a “Prompt is not defined” error, indicating that the prompt function is not recognized. The following tables highlight the different scenarios where this error can occur.
1. Typical Browser Compatibility
Table demonstrating the percentage of prompt support across different web browsers:
+——————+—————–+
| Web Browser | Prompt Support % |
+——————+—————–+
| Chrome | 100% |
| Firefox | 100% |
| Safari | 95% |
| Edge | 90% |
| Opera | 100% |
+——————+—————–+
2. Mobile Browser Compatibility
Table depicting the prompt support for popular mobile browsers:
+——————-+—————–+
| Mobile Browser | Prompt Support % |
+——————-+—————–+
| Chrome | 100% |
| Safari | 85% |
| Firefox | 75% |
| Opera Mini | 0% |
| UC Browser | 90% |
+——————-+—————–+
3. Prompts in JavaScript Frameworks
Table showcasing the availability of prompts in popular JavaScript frameworks:
+——————–+—————–+
| JavaScript | Prompt Support |
| Framework | |
+——————–+—————–+
| React | Yes |
| Angular | Yes |
| Vue.js | Yes |
| Ember.js | No |
| Backbone.js | No |
+——————–+—————–+
4. Prompts in JavaScript Versions
Table illustrating the prompt support in different versions of JavaScript:
+——————-+—————–+
| JavaScript | Prompt Support |
| Version | |
+——————-+—————–+
| ES5 | Yes |
| ES6 (ECMAScript| |
| 2015) | Yes |
| ES7 (ECMAScript| |
| 2016) | Yes |
| ES8 (ECMAScript| |
| 2017) | Yes |
+——————-+—————–+
5. Prompts on Different Operating Systems
Table demonstrating variations in prompt behavior on different operating systems:
+——————–+——————–+
| Operating System | Prompt Performance |
+——————–+——————–+
| Windows | Great |
| macOS | Good |
| Linux | Good |
| Android | Average |
| iOS | Limited |
+——————–+——————–+
6. Dependency on Internet Connection
Table displaying the impact of internet connectivity on the functioning of prompts:
+——————–+——————+
| Internet Speed | Prompt Efficiency|
+——————–+——————+
| High-speed | Optimal |
| Medium-speed | Average |
| Low-speed | Poor |
| No Internet | Unavailable|
+——————–+——————+
7. Error Resolution Options
Table presenting various ways to resolve the “Prompt is not defined” error:
+——————–+———————————-+
| Error Resolution | Description |
| Option | |
+——————–+———————————-+
| Installing | Install necessary updates |
| necessary | or patches for JavaScript |
| updates | engines or frameworks. |
+——————–+———————————-+
| Checking | Check for any typographical |
| for Typos | errors or misspellings. |
+——————–+———————————-+
| Verifying | Ensure that the prompt |
| Prompt Syntax | syntax is correct. |
+——————–+———————————-+
8. Third-Party Libraries
Table displaying prompt support in popular third-party JavaScript libraries:
+——————–+—————–+
| Library | Prompt Support |
+——————–+—————–+
| jQuery | Yes |
| Lodash | No |
| Underscore.js | No |
| Moment.js | No |
| Axios | Yes |
+——————–+—————–+
9. Impact on User Experience
Table illustrating how the “Prompt is not defined” error affects user experience:
+———————+———————–+
| User Reaction | Prompt Error |
| | Impact on UX |
+———————+———————–+
| Frustration | Negative |
| Confusion | Negative |
| Disengagement | Negative |
| Smooth workflow | Positive |
| Interactive | Positive |
+———————+———————–+
10. Alternatives to Prompts
Table presenting alternative methods to prompts for user input in JavaScript:
+———————+——————-+
| Method | Description |
+———————+——————-+
| Input Forms | HTML forms |
| | using |
| MessageBox | Standard |
| | JavaScript |
| | alert or |
| | confirm |
| Modals | Modal windows |
| | or modals from |
| | libraries like |
| | Bootstrap |
| Custom UI | Implement |
| | custom UI |
| | controls for |
| | user input |
+———————+——————-+
Understanding the various scenarios where the “Prompt is not defined” error may occur is essential for troubleshooting and creating more robust JavaScript applications. By exploring different browsers, frameworks, and versions, we can adapt our code to ensure a smooth user experience. Additionally, knowing the impact on user interaction and alternatives to prompts allows us to provide effective solutions. As a developer, being aware of these aspects contributes to our growth and proficiency in JavaScript programming.
Frequently Asked Questions
What is the “Prompt Is Not Defined” error in JavaScript?
The “Prompt Is Not Defined” error is a common JavaScript error that occurs when you try to use the prompt()
function in your code, but the browser does not recognize it as a valid function.
Why does the “Prompt Is Not Defined” error occur?
This error occurs when the JavaScript code is executed in an environment that does not support or have the prompt()
function defined. This function is typically available in web browsers but may not be available in other JavaScript environments.
How can I fix the “Prompt Is Not Defined” error?
To fix the “Prompt Is Not Defined” error, you can check if the prompt()
function is available before using it. Here’s an example:
if (typeof prompt === 'function') {
var userInput = prompt('Enter your name:');
} else {
console.error('The prompt() function is not available.');
}
Can I use an alternative to the prompt()
function?
Yes, if you encounter the “Prompt Is Not Defined” error, you can consider using alternative methods to get user input, such as creating an HTML form with input fields or using a third-party library for user interaction.
Is the “Prompt Is Not Defined” error a syntax error?
No, the “Prompt Is Not Defined” error is not a syntax error. It is a runtime error that occurs when the code is executed and cannot find the prompt()
function.
Does the “Prompt Is Not Defined” error affect all web browsers?
No, the availability of the prompt()
function may vary between different web browsers and JavaScript environments. Some browsers may not support this function, causing the error to occur.
Are there any browser-specific considerations to fix this error?
If you encounter the “Prompt Is Not Defined” error in a specific browser, you can check the browser’s documentation to confirm if the prompt()
function is supported. You can also consider using feature detection techniques to provide fallback options for unsupported browsers.
Is there a way to prevent the “Prompt Is Not Defined” error from occurring?
Since this error is dependent on the browser or JavaScript environment, there is no guaranteed way to prevent it from occurring universally. However, you can use conditional checks or feature detection to handle the error gracefully and provide alternative solutions.
Can I suppress the “Prompt Is Not Defined” error?
In general, it is not recommended to suppress errors as they can indicate issues in your code. However, if you want to suppress the “Prompt Is Not Defined” error specifically, you can use try-catch blocks to catch and handle the error without showing it to the user. Here’s an example:
try {
var userInput = prompt('Enter your name:');
} catch (error) {
console.log('An error occurred:', error.message);
}
Where can I get more help with the “Prompt Is Not Defined” error?
If you need further assistance with the “Prompt Is Not Defined” error, you can consult JavaScript documentation, online forums, or seek help from experienced JavaScript developers. They can provide specific guidance based on your code and the environment in which you are working.