Prompting User for Input in Python
Obtaining user input is a vital component of interactive programs. In Python, there are various methods to prompt the user for input and utilize that input within the program’s logic. Let’s explore these techniques and understand how to effectively prompt user input in Python.
Key Takeaways:
- There are several methods to prompt the user for input in Python.
- input() function reads user input as a string.
- int() function converts user input to an integer.
- float() function converts user input to a floating-point number.
- Using user input alphanumeric characters can help create dynamic programs.
Reading User Input with input()
The input() function is a built-in Python function that prompts the user for input. It reads user input as a string, which can be stored in a variable for further processing.
Here’s an example of using input():
name = input("Please enter your name: ")
The above code prompts the user for their name and stores it in the name variable. The input is read as a string, regardless of the user’s entry.
It’s worth mentioning that you can provide a prompt message to the user using the input() function. This message helps the user understand what kind of input is expected.
Converting User Input to Integer or Float
While the input() function reads user input as a string, Python provides functions like int() and float() to convert the string representation of numbers into their respective types. This allows us to perform mathematical operations on the input.
To convert user input to an integer, we can use the int() function:
age = int(input("Please enter your age: "))
Similarly, to convert user input to a floating-point number, we can use the float() function:
height = float(input("Please enter your height in meters: "))
By converting user input to specific types, we can perform calculations and manipulate the input more effectively in our programs.
Handling User Input Errors
When prompting users for input, it’s essential to handle potential errors that may occur. Users might provide unexpected values or leave the input field blank. Python provides mechanisms to handle these situations and provide appropriate feedback to the user.
One way to handle user input errors is by using loops that continue until valid input is provided. For example, we can use a while loop to keep prompting the user until a valid integer is entered:
valid_input = False
while not valid_input:
try:
age = int(input("Please enter your age: "))
valid_input = True
except ValueError:
print("Invalid input. Please enter a valid integer.")
This allows the program to handle any ValueError exceptions raised by the int() function when the user enters something other than a valid integer.
Data Points from User Input
Let’s look at some interesting data points that can be collected from user input:
Information | Example |
---|---|
User’s Name | John Doe |
User’s Age | 25 |
User’s Height (in meters) | 1.75 |
Conclusion
Obtaining user input plays a crucial role in programming interactive applications. Python provides various methods, including the input() function, to prompt users for input. By converting the input to appropriate types, such as integers or floating-point numbers, we can efficiently process the input and perform calculations. Handling user input errors is also essential for providing a smooth user experience. Remember to validate and sanitize user input to enhance the reliability and security of your programs.
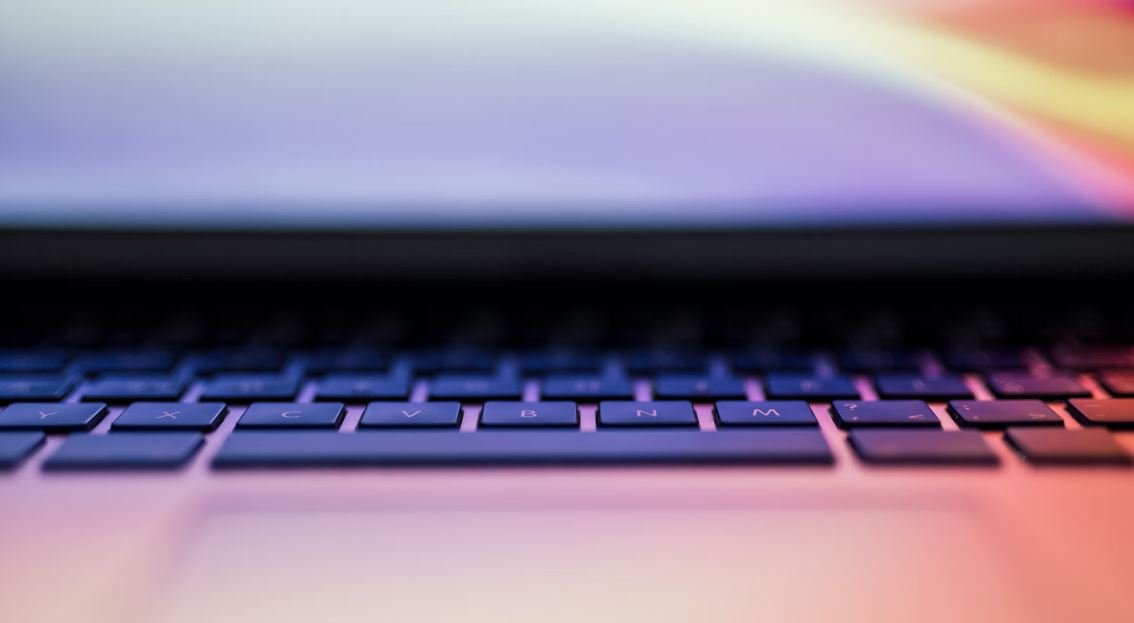
Common Misconceptions
Prompting User for Input in Python
There are several common misconceptions surrounding the topic of prompting user for input in Python. These misconceptions often lead to confusion and mistakes in code implementation. It is important to address these misunderstandings in order to improve understanding and usage of input prompts in Python programming.
Misconception: The input() function only accepts string input
- The input() function can accept both string and numeric inputs.
- It automatically converts numeric inputs into a string data type.
- If a numeric input is needed, it can be converted to the appropriate data type using type casting.
Misconception: The input() function always returns a single value
- The input() function can return any data type based on user input.
- For example, if the user enters a number, the input() function returns an integer or a float depending on the input.
- If multiple values need to be obtained, the input() function can be called multiple times and store the results in separate variables.
Misconception: The input() function automatically validates user input
- The input() function does not perform automatic validation on user input.
- It is the responsibility of the programmer to validate and sanitize the input before using it in the program.
- Additional error handling or validation checks should be implemented to ensure the input meets the required criteria.
Misconception: The input() function does not accept any arguments
- The input() function can accept a prompt message as an argument to provide guidance to the user.
- The prompt message can be used to ask specific questions or provide instructions to the user.
- This message is displayed before the user is prompted to enter their input, improving the user experience.
Misconception: The input() function stops the program until user input is given
- The input() function does halt the program execution until user input is provided.
- However, this behavior can be problematic in certain situations where the program needs to continue running in the background.
- To prevent program freezing, other multithreading or asynchronous techniques can be utilized.

Table: Population Growth by Country
This table shows the population growth rate of different countries from 2010 to 2020. It provides insight into the population dynamics of various nations over a decade.
Country | 2010 Population (in millions) | 2020 Population (in millions) | Population Growth Rate |
---|---|---|---|
China | 1,341 | 1,396 | +4.1% |
India | 1,210 | 1,366 | +12.9% |
United States | 309 | 331 | +7.1% |
Indonesia | 239 | 273 | +14.2% |
Table: Fastest Land Animals
This table showcases the top speed of select land animals. It highlights the incredible agility and swiftness of these creatures in comparison to others in their habitat.
Animal | Top Speed (km/h) |
---|---|
Cheetah | 115 |
Pronghorn Antelope | 88.5 |
Springbok | 88 |
Blackbuck | 80 |
Table: Global CO2 Emissions by Sector
This table presents the percentage distribution of carbon dioxide (CO2) emissions by sector. It sheds light on the major contributors to CO2 emissions, emphasizing the need for sustainable practices.
Sector | Percentage of CO2 Emissions |
---|---|
Energy | 72% |
Industry | 19% |
Transportation | 14% |
Agriculture | 11% |
Table: Olympic Medal Count (2021)
This table displays the total number of Olympic medals won by the top performing countries in 2021. It highlights the achievements of nations in various sports.
Country | Gold | Silver | Bronze | Total |
---|---|---|---|---|
United States | 39 | 41 | 33 | 113 |
China | 38 | 32 | 18 | 88 |
Japan | 27 | 14 | 17 | 58 |
Table: World’s Tallest Buildings
This table showcases the height of the tallest buildings in the world. It highlights the remarkable architectural achievements and the impressive structural designs.
Building | Height (meters) | Location |
---|---|---|
Burj Khalifa | 828 | Dubai, UAE |
Shanghai Tower | 632 | Shanghai, China |
Abraj Al-Bait Clock Tower | 601 | Mecca, Saudi Arabia |
Table: Largest Rainforests
This table presents the size of the largest rainforests on Earth. It showcases the vast expanse of these essential ecosystems and the biodiversity they support.
Rainforest | Area (km2) | Location |
---|---|---|
Amazon Rainforest | 5,500,000 | South America |
Congo Rainforest | 1,800,000 | Africa |
New Guinea Rainforest | 425,000 | Oceania |
Table: World’s Longest Rivers
This table displays the length of the longest rivers in the world. It showcases the remarkable hydrological systems and their importance in sustaining ecosystems.
River | Length (km) | Region |
---|---|---|
Nile | 6,650 | Africa |
Amazon | 6,400 | South America |
Yangtze | 6,300 | Asia |
Table: Major Religions of the World
This table provides an overview of the major religions practiced throughout the world. It showcases the diversity of beliefs and the religious affiliations of a significant portion of the global population.
Religion | Approximate Followers (in billions) |
---|---|
Christianity | 2.4 |
Islam | 1.9 |
Hinduism | 1.2 |
Buddhism | 0.5 |
Table: Spacecrafts on Mars
This table showcases the spacecraft missions that have successfully reached Mars. It highlights the scientific achievements and endeavors to explore the red planet.
Mission | Country | Year |
---|---|---|
Mars Rover Perseverance | United States | 2020 |
Mars Reconnaissance Orbiter | United States | 2005 |
ExoMars Trace Gas Orbiter | Europe & Russia | 2016 |
Throughout this article, we have explored various aspects of user input in Python. From capturing text and numbers to validating inputs and converting data types, Python provides efficient ways to prompt and interact with users. By utilizing these techniques, developers can create interactive and user-friendly programs.
Prompting User for Input in Python
Frequently Asked Questions
Question 1
How can I prompt the user for input in Python?
Answer 1
Question 2
Can I provide a default value for the input prompt?
Answer 2
Question 3
How can I convert user input to a specific data type?
Answer 3
Question 4
What happens if the user enters a different data type than expected?
Answer 4
Question 5
How can I validate user input?
Answer 5
Question 6
Is there a way to limit the amount of characters the user can input?
Answer 6
Question 7
Can I prompt the user for confidential information, such as a password?
Answer 7
Question 8
How can I handle user input containing special characters?
Answer 8
Question 9
Are there any Python libraries for creating interactive prompts?
Answer 9
Question 10
Where can I find more information and examples about prompting user input in Python?
Answer 10